Input devices are often called Sensors. Output devices are often called Actuators. The process of connecting a Sensor or Actuator to a computer processor system is called Interfacing.
Sensors
- A sensor is a device, module, machine or subsystem whose purpose is to detect events or changes in its environment and send the information to other electronics, frequently a computer processor. (Ref: Wikipedia: Sensor)
- Used in everyday objects to read changes in the environment.
- Sensor readings are usually analog by nature.
- Changes are usually slower in availability (compared to computer processors) and require some processing techniques.
Methods of reading sensors
- There are 3 basic methods of reading/obtaining information from a sensor. These methods are dependent upon the availability of the information, as read by the sensor
- Always available
- Just read the data, data is always available
- Changes to the reading occur very slowly.
- E.g. temperature
- Polling
- Processor has to continually query/poll the sensor for information.
- Sensor will usually indicate whether there is new information through another signal or have a drastic change in the reading to indicate that there is a new reading available.
- When Processor “sees” this change, data is read.
- E.g. switch
- Triggering
- A trigger is a signal(s) that is used to initiate the reading.
- Sequence is as follows:
- Processor sends a signal to the sensor for data
- Sensor gathers information, and when ready
- Sends reading back to the Processor. The Sensor may also indicate that data is ready to be read by sending back an Acknowledge/Ready signal
- E.g. Ultrasonic distance measurement
- Sensor data
- Real environment data is analog by nature. It may be some physical element e.g. temperature, distance
- A sensor converts this data into an equivalent electrical signal/voltage that is sent to the computer processor.
- The computer processor has to read this electrical signal and convert it into an understandable value.
- Analog signals
- most common type of sensor data
- requires conversion to a digital value, usually with an ADC
- usually it is a direct relationship e.g. V = K * environment-value
- Digital signals
- these sensors are configured as smart devices, with processing power
- sensor itself converts the analog values into a digital signal (e.g. pulse or series of digits)
- processor needs to decode to understand the value
- Arduino Libraries
- The Arduino System is very popular because there is large number of libraries (of code) available for almost every Sensor that you can think of.
- Add libraries to the Arduino IDE System (Ref: Arduino.cc Installing Additional Arduino Libraries)
- There are two types of libraries,
- Search able using the Arduino IDE system (Internal)
- External libraries, available as compressed C++ code.
- How to add a library using the Library Manager
IDE > Sketch > Install Library > Manage Libraries
- Search for the library
- Highlight and click Install, when complete, it should state “Installed”
- How to add an External library (.ZIP format)
- Search for the library on the Internet
- Download the library, usually in a compressed .ZIP file
IDE > Sketch > Include Library > Add .Zip Library
- Close the IDE. Re-open.
- How to manually add an External Library
- Search for the library on the Internet
- Download the library, usually in a compressed .ZIP file
- Locate where your sketch folder is found on your computer using
IDE > File > Preferences > Sketchbook
- Extract the Library from the ZIP file (this is usually a folder)
- Copy the extracted library folder to [Sketchbook folder] > Libraries folder
- Close the IDE. Re-open.
- Check that the Library has been added using
IDE > Sketch > Include Library
. You should see your installed library there.
- To apply the library code to your application, add the header file a the top of your sketch using
IDE > Sketch > Include Library > LibName
. The header file has an extension of .h
.
Types of Sensors
- Switches
- An electrical component that can disconnect or connect the conducting path of a circuit.
- Provides a LOW or HIGH signal depending on the circuitry.
- Use
digitalRead()
to determine value of a switch.
- Be aware of debouncing techniques when mechanical switches are in use.
- Types of switches
- Toggle
- a mechanical switch that changes the state each time the switch is thrown/manipulated.
- e.g. If in the ON position, throwing the switch will change it to an OFF position.
- the state remains until the switch is thrown
- Pushbutton switch
- a spring-loaded mechanical switch that changes state when the switch is pressed. When released (and because of the spring) the switch returns to the original state.
- Two types of pushbutton switches
- normally closed - push to open/break connection
- normally open - push to close/make connection
- Slide switch
- a switch which is closed or opened by a sliding mechanism.
- when changed, the switch remains in the new state.
- Touch Capacitative switch
- switch works based on body capacitance.
- when a person touches it, the body increases the capacitance of the switch and triggers the switch
- Temperature, Humidity
- the environment can be measured using a number of devices, the most common and cheapest is to use the DHT-11 temperature and humidity sensor.
- temperature is a physical quantity that expresses hot or cold.
- reference temperatures
- freezing point of water = 0 degrees Centigrade
- boiling point of water = 100 degrees Centigrade
- typical Singapore temperature = 28 - 32 degrees Centigrade
- humidity is the measure of the concentration of water vapor present in the air. Singapore’s humidity is between 70-90%.
- the following devices can be used with the Arduino system
- DHT-11
- operating voltage range 3.5~5.5V
- temperature range 0-50C, humidity 20-90%
- resolution 16-bit
- accuracy +/- 1C, +/- 1%
- DHT-22
- temperature range -40~80C, humidity 0~100%
- resolution 16-bit
- accuracy +/- 0.5C, +/- 1%
- LM35 temperature sensor
- operating voltage range -2~35V, typical 5V
- temperature range -55C ~ 150C
- accuracy +/- 0.5C
- DS18B20 temperature probe
- operating voltage range 3V ~ 5V
- temperature range -55C ~ 125C
- accuracy +/- 0.5C
- Interfacing to the Arduino Uno
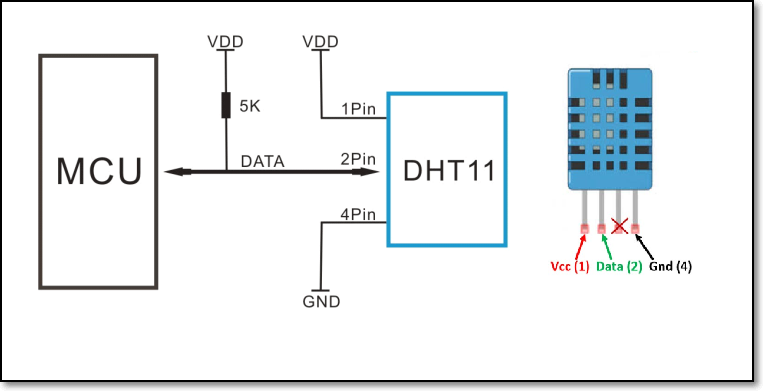
- Distance
- This depends on the distance to be measured. The devices can range from a limit switch to an ultrasonic sensor.
- Photoelectric InfraRed Avoidance detection/Proximity sensor
- distance: 2cm ~ 40cm
- can use ENable pulse or continuous measurement
- avoidance distance is adjusted with potentiometer
- does not measure distance, just detects it
- Ultrasonic HC-SR04
- distance: 2cm ~ 4 m
- uses SONAR, returns a pulse proportional to the distance
- ranging accuracy 3mm, measuring angle 15 degrees
- distance is equivalent to pulse measured
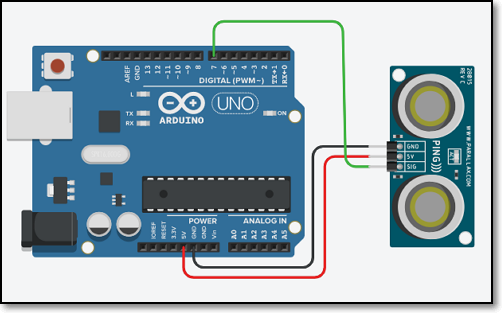
- Motion detection
- Commonly used to detect the presence of humans or animals in a room/vicinity.
- HC-SR501 PIR Sensor
- PIR - Passive Infra Red Sensor
- Detectable cover distance 120 degrees, 7 meters
- has a repeatable and non-repeatable operating mode
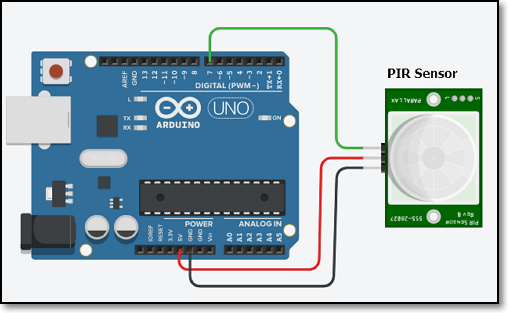
- Light Measurement & Detection
- Light intensity can be detected/measured using LDR (Light Dependent Resistors) or Light Sensor Modules (using Photo transistor circuits)
- LDR 5516
- Low cost measurement/detection of light using LDR
- Variable analog voltage received as input\
- Can be calibrated
- Measured using Arduino analogRead() function
- Use a 5K ~ 10K resistor for the Arduino Uno
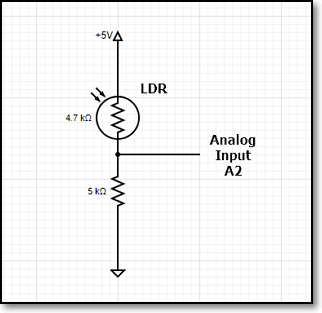
- Time
- These are modules that allow you to keep track of the time. A RTC uses a small battery, crystal and memory to maintain a on-going clock which can be read to provide the current date and time.
- Real-time Clock Modules
- Rotary Encoders
- a rotary encoder reads the positional value of a shaft by comparing 2 pulses as the shaft turns.
- looks like a potentiometer, but does not change resistance
- rotary encoders are now used for volume controls, motor encoders etc
- Weight
- A load cell provides a means of measuring the weight of an object. This is done by the measuring the contraction of metals. Usually a module is provided to read the data from a load cell and transfer the results to the computer processor.
- Load Cell Weight Sensor HX711 AD Converter
- Water
In this assignment, you will attempt to interface an input analog device to your Arduino and produce equivalent output on a RGB LED.
«««< HEAD:input_devices/input_devices.md
You can find the assignment here
Useful sites for Arduino Interfacing
Here are some of the more “reputable/trust-worthy” sites for Arduino modules
You can view the assignment here
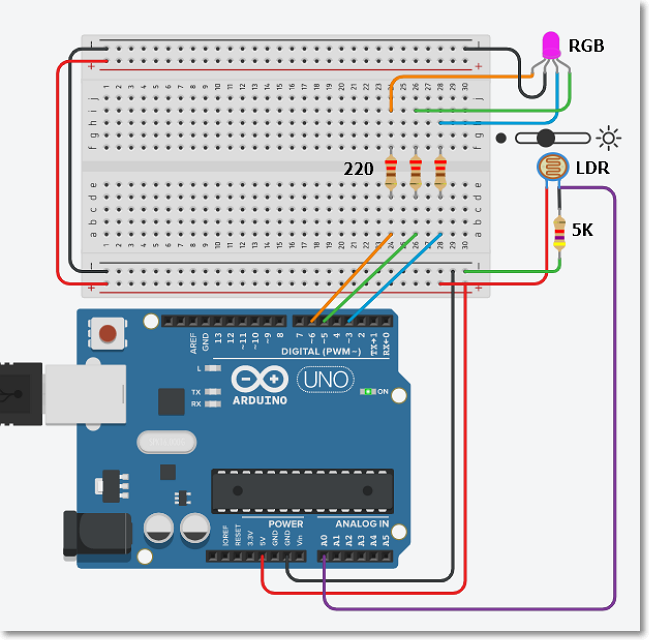
d5ae412f2f86efb3fc30523253ebbd20ce1a1de9:input_devices/inputdevices.md
January 2021